Using OpenSCAD to model 3D structures
|
Building a Plane
A model airplane also consists of basic elements. A wing is made when you create a separate module and name it wing . A module is similar in principle to a function. It accepts parameter and delivers an object in return, in this case a wing (Listing 2, line 24).
Listing 2
An Airplane
01 $fn=100; 02 sep() airplane(); 03 04 module airplane(){ 05 // Wings 06 wing(0.7); 07 translate([18,0,0]) wing(1); 08 translate([0,0,2.1]) rotate([90,-20,0]) wing(0.45); 09 // Hull 10 hull(){ 11 translate([-3,0,0]) sphere(1.5); 12 translate([24,0,0]) sphere(3); 13 }; 14 // Cockpit 15 translate([19,0,2]) scale([2,1,1]) color("red",0.6) sphere(2); 16 //Propeller 17 translate([26.5,0,0]) scale([2,1,1]) color("grey") sphere(1); 18 translate([27.3,0,0]) rotate([0,90,0]) color("grey",0.5) cylinder(h=0.5, r=5); 19 // Wing Module 20 module wing(s=1){ 21 rotate([90,0,0]) 22 scale([3*s,0.4*s,1*s*s]) 23 cylinder(h=35,r=1,center=true); 24 }; 25 }; 26 27 module sep(){ 28 translate([0,0,-0.5]) difference(){ 29 children(); 30 translate([-40,-40,0]) cube(100); 31 }; 32 translate([0,0,0.5]) intersection(){ 33 children(); 34 translate([-40,-40,0]) cube(100); 35 }; 36 };
The curly braces are necessary because a module typically contains more than one object. Here, I will show how to make the wings from a deformed cylinder.
scale([3,0.4,1]) cylinder(h=35,r=1)
The command stretches the X axis by a factor of 3, compresses the Y axis by a factor of 0.4, and leaves the Z axis alone. The call wing(1) creates the hydrofoils, wings(0.7) the elevator assembly. The translate() command, which is found in the script as a prefix, moves the components into the correct position.
The fuselage here consists of two spheres – one in front and one in back. The hull() command encircles both objects with a shell and connects them to a new object. You should add the other elements according to the pattern presented above (Figure 5).
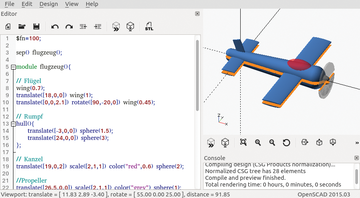
One way to print the model with a 3D printer is to divide the object into two parts to achieve a smooth bottom layer. The module sep() performs the separation. The object given by children() , or child() in case you are using a pre 2015 version, is the object that sep() works on. The difference() command subtracts the upper part of the airplane and intersection() precisely describes the space shared in common with the cube.
Modularizing Code
The program is more manageable when you store the main part of the program in its own file. An example using Lego bricks can clarify this method. Building these bricks functions just like the construction of the airplane from individual geometric objects. It is tiresome to measure and place the elements, so I relied on one of the numerous libraries for assistance [2].
Given the file name for the Lego bricks data lego_brici_builder.scad , then two lines are enough to display one 6x2 brick.
use <lego_brici_builder.scad> brick(6,2,3);
Additional commands can even make it possible to build a tower. The loop for (i=[0:1:6]) iterates the variable i from 0 up to 6 inclusively in increments of one. Because the loop contains only one object, curly braces are not required. The rotate() and translate() commands rotate and raise the bricks with each iteration (Figure 6).
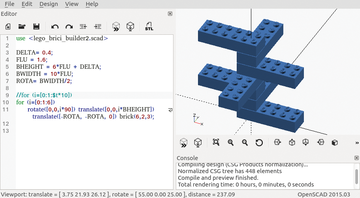
If you would like to look at the construction of the bricks, replace the number 6 in the loop definition by the variable $t*10 and select the menu option View | animate . This creates a new input line underneath the output window. In this line, enter the number 10 for Steps and a 1 for FPS (frames per second) .
The internal variable $t rises by increments of one tenth every second with these settings. Once it reaches 1 , it will start over again with 0 . The loop rebuilds the tower every second, each time just a bit more of it. Images are saved when you activate the checkbox on the right next to the Steps field.
A program like Ffmpeg makes a video of the images. Because the software controls the position of the observer via an internal variable, it is even possible to take tracking shots.
Leaving keys lying about, even in digital image form can create security problems. In this example, I demonstrate this state of affairs with the image of a key found on Wikimedia Commons [3]. I used a straightforward digitizing program to define 25 reference points on the key bit. These values function as the vector with the 2D points serving as components. You should convert the points into a polygon chain via the following command. Afterward, you can convert the chain into a key bit that has a thickness of 0.2 centimeters:
linear_extrude(height=0.2) polygon(points);
Four additional commands via difference() cut two slots in the upper side and two slots into the underside of the key. Then, comes a ring for holding the key and the 3D print template is ready to use (Figure 7).
« Previous 1 2 3 4 Next »
Buy this article as PDF
Pages: 6
(incl. VAT)